When FlutterFlow introduced Libraries, we at Appwrite saw an exciting opportunity to create a powerful integration that would make secure authentication accessible to FlutterFlow users. This article takes you behind the scenes of how we built the Appwrite Authentication Library and the technical decisions that shaped its development.
Why We Built the Appwrite FlutterFlow Library
Appwrite is a secure, open-source backend server that provides developers with a comprehensive set of APIs for building web, mobile, and Flutter applications. Our goal was to make Appwrite's robust authentication system easily accessible to FlutterFlow users, allowing them to implement secure user authentication without writing complex code.
The Libraries feature provided the perfect platform for this integration. It allowed us to create a centralized source of reusable resources that could be shared across multiple FlutterFlow projects, making authentication implementation as simple as drag-and-drop.
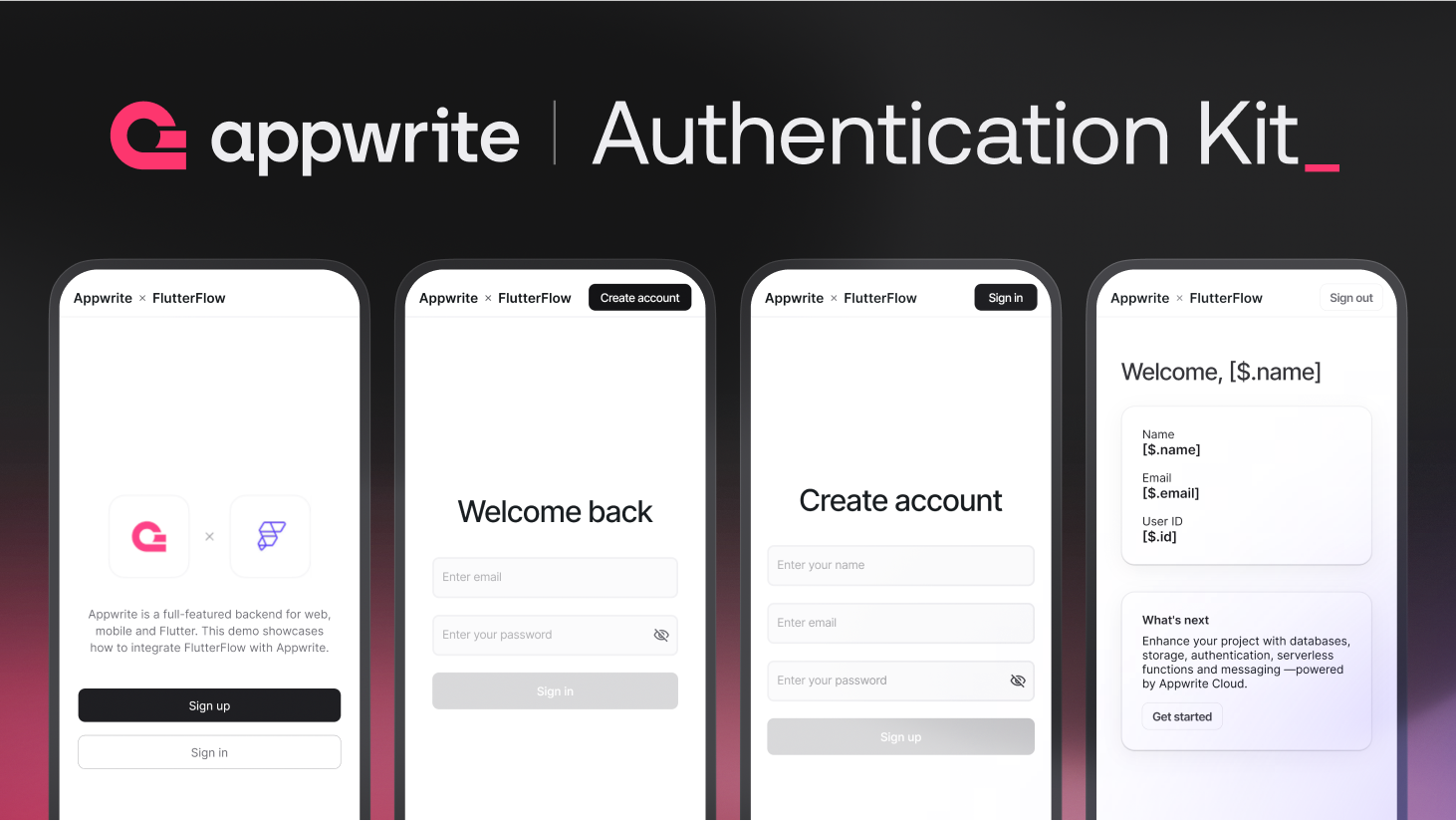
The Technical Implementation
1. Core Architecture
The library's architecture is built around several key components, combining FlutterFlow's capabilities with custom logic written by Appwrite:
- Custom Actions: Built by Appwrite to wrap around Appwrite’s Flutter SDK for core auth flows.
- App States: FlutterFlow’s built-in state management system, used to store and persist user session data.
- Custom Data Types: Implemented by Appwrite for type safety and structure (e.g.,
AppwriteUser
). - Error Handling System: Custom logic by Appwrite to show user-friendly error messages and logs.
class AppwriteAuthLibrary {
final AppwriteConfig config; // Appwrite
final AppwriteUserState userState; // FlutterFlow App State
final Map<String, AuthActionHandler> actions; // Appwrite
final AppwriteErrorHandler errorHandler; // Appwrite
}
Core Library Structure
2. SDK Integration
The first technical challenge was integrating our existing Flutter SDK with FlutterFlow's visual builder:
A. Dependency Management (by Appwrite)
- Added
appwrite
as a project dependency. - Version constraints and compatibility checks.
- Semantic versioning strategy for predictable updates.
B. Configuration Management
FlutterFlow provides secure storage as part of its generated architecture. Appwrite added logic to securely store and validate config data (like endpoint, project ID, and API key) using this storage.
class AppwriteConfig {
final String apiEndpoint;
final String projectId;
final String apiKey;
// Secure storage implementation
Future<void> saveConfig() async {
await SecureStorage.write('appwrite_config', {
'apiEndpoint': apiEndpoint,
'projectId': projectId,
'apiKey': apiKey,
});
}
}
Configuration Management Example
3. Data Type System
One of the most interesting technical challenges was creating a type-safe system that works seamlessly with FlutterFlow:
A. Custom Data Types
Implemented by Appwrite:
- Created
AppwriteUser
andAppwriteUserResponse
types. - Added conversion logic between Appwrite SDK response and FlutterFlow-compatible structures.
- Ensured validation and runtime type safety.
class AppwriteUser {
final String id;
final String email;
final String name;
final bool emailVerified;
final String status;
// Type conversion methods
Map<String, dynamic> toJson() {
return {
'id': id,
'email': email,
'name': name,
'emailVerified': emailVerified,
'status': status,
};
}
// Validation methods
bool isValid() {
return id.isNotEmpty && email.isNotEmpty;
}
}
Custom Data Type Example
B. State Management
One of the most important aspects was handling user sessions and state. FlutterFlow provides a built-in App State system, which we used to persist data across app restarts.
What we implemented:
- Leveraged FlutterFlow’s App State system for reactive updates.
- Added custom logic to update, clear, and sync session state.
- Implemented error handling and fallback strategies when state becomes invalid.
class AppwriteUserState {
AppwriteUser? currentUser;
void update(AppwriteUser user) {
currentUser = user;
}
void clear() {
currentUser = null;
}
}
So in conclusion:
- We implemented a reactive state system which leverages FlutterFlow's App State with custom hooks added by Appwrite.
- Built error state handling and recovery mechanisms which was implemented by Appwrite via custom code & Action Flow.
- Added state synchronization across app restarts powered by FlutterFlow’s persistent App State.
4. Action Implementation
The heart of the library lies in its custom actions:
A. Authentication Actions
initialize
: Sets up the SDK and validates configurationsignUpWithEmailAndPassword
: Handles user registrationsignInWithEmail
: Manages user loginsignOut
: Handles session terminationgetCurrentUser
: Provides session state management
class SignInAction extends AuthAction {
@override
Future<AppwriteUserResponse> execute(Map<String, dynamic> params) async {
try {
final email = params['email'] as String;
final password = params['password'] as String;
// Validate input
if (!isValidEmail(email)) {
return AppwriteUserResponse.error('Invalid email format');
}
// Execute sign in
final result = await appwrite.account.createSession(
email: email,
password: password,
);
// Update state
await userState.update(result);
return AppwriteUserResponse.success(result);
} catch (e) {
return AppwriteUserResponse.error(
errorHandler.formatError(e),
);
}
}
}
Action Implementation Example
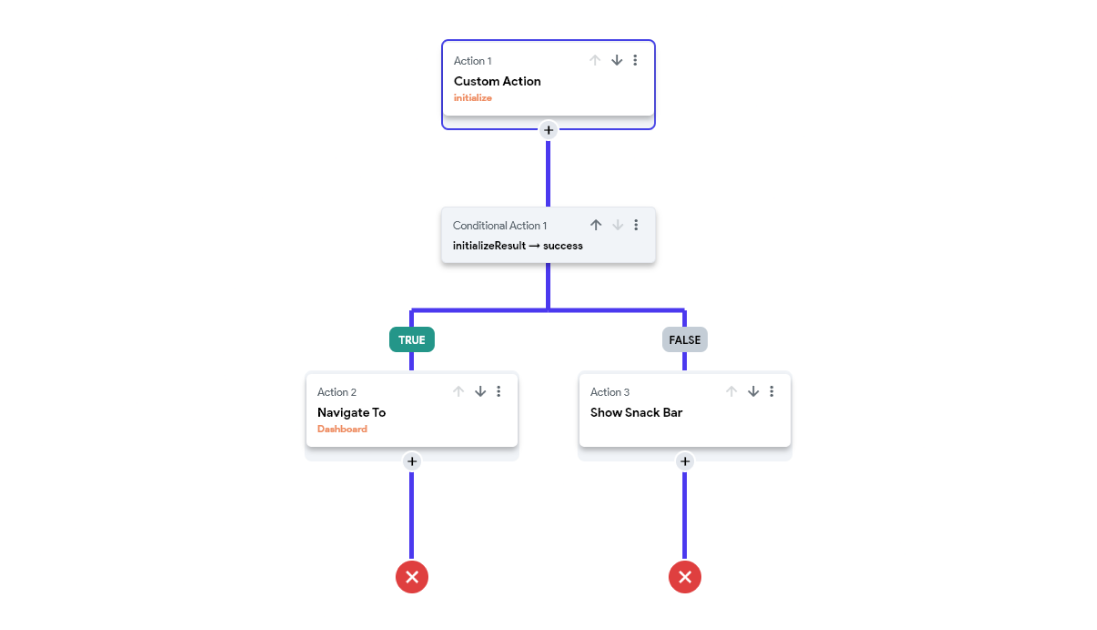
B. Error Handling
- Implemented a consistent error response format
- Created user-friendly error messages
- Built logging for debugging and monitoring
- Added error recovery mechanisms
Testing the Library
To test the Appwrite FlutterFlow Library, we created a sample project that imports the Library as a dependency. During active development, we used the "current" version rather than a static version to ensure we were always testing the latest changes.
This sample project served two important purposes:
1. Testing the complete authentication flow from sign-up to session management
2. Providing a public demo for the community to understand how to use the library
The testing process helped us identify and fix several important issues:
- Configuration validation
- Error handling in different scenarios
- State management across app restarts
- Platform-specific behaviors
Creating Documentation
Once the Library was thoroughly tested, we created comprehensive documentation to guide users through the implementation process. The documentation covers:
- Step-by-step setup instructions
- Configuration requirements
- Common use cases and examples
- Troubleshooting guides
- Platform-specific considerations
What's Next
With FlutterFlow's Marketplace support for Libraries, we're excited to make the Appwrite Library more accessible to FlutterFlow users.
The Marketplace integration allows developers to:
- Easily discover the Appwrite Library
- Access our documentation
- Add the library to their projects with a single click
As our SDK evolves, the library will be updated to incorporate new features and improvements. With robust version control in place, we can seamlessly upgrade the package dependency version in our Library project, test new capabilities, and publish updates for FlutterFlow users.
Conclusion
Building the Appwrite FlutterFlow Library was a challenging but rewarding experience. It allowed us to make our authentication system accessible to a broader audience while learning valuable lessons about creating developer tools.
We're excited to see how developers use this library to build secure applications with FlutterFlow.
If you're interested in building your own FlutterFlow library, we encourage you to start with a clear problem to solve and focus on making it as simple and flexible as possible. The FlutterFlow team has created an excellent platform for sharing and distributing libraries, and we're excited to see what the community builds next.
More Resources
- Appwrite Documentation
- FlutterFlow Marketplace
- Appwrite FlutterFlow Demo App